Start tracking subscribers in AWS in under 30 minutes
Building an audience is key to growing your brand. You need a way to keep them informed! This tutorial will have you tracking your subscribers in AWS in no time.
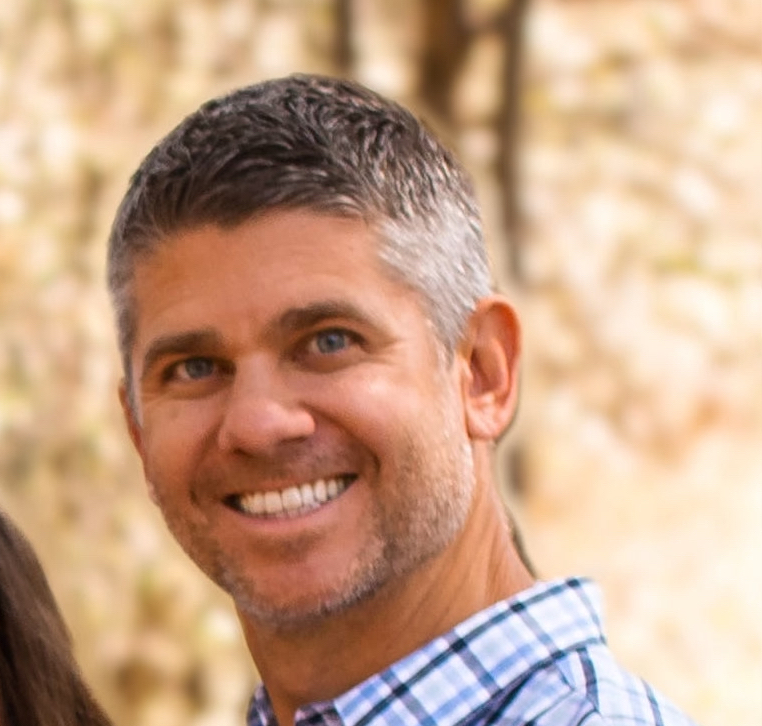
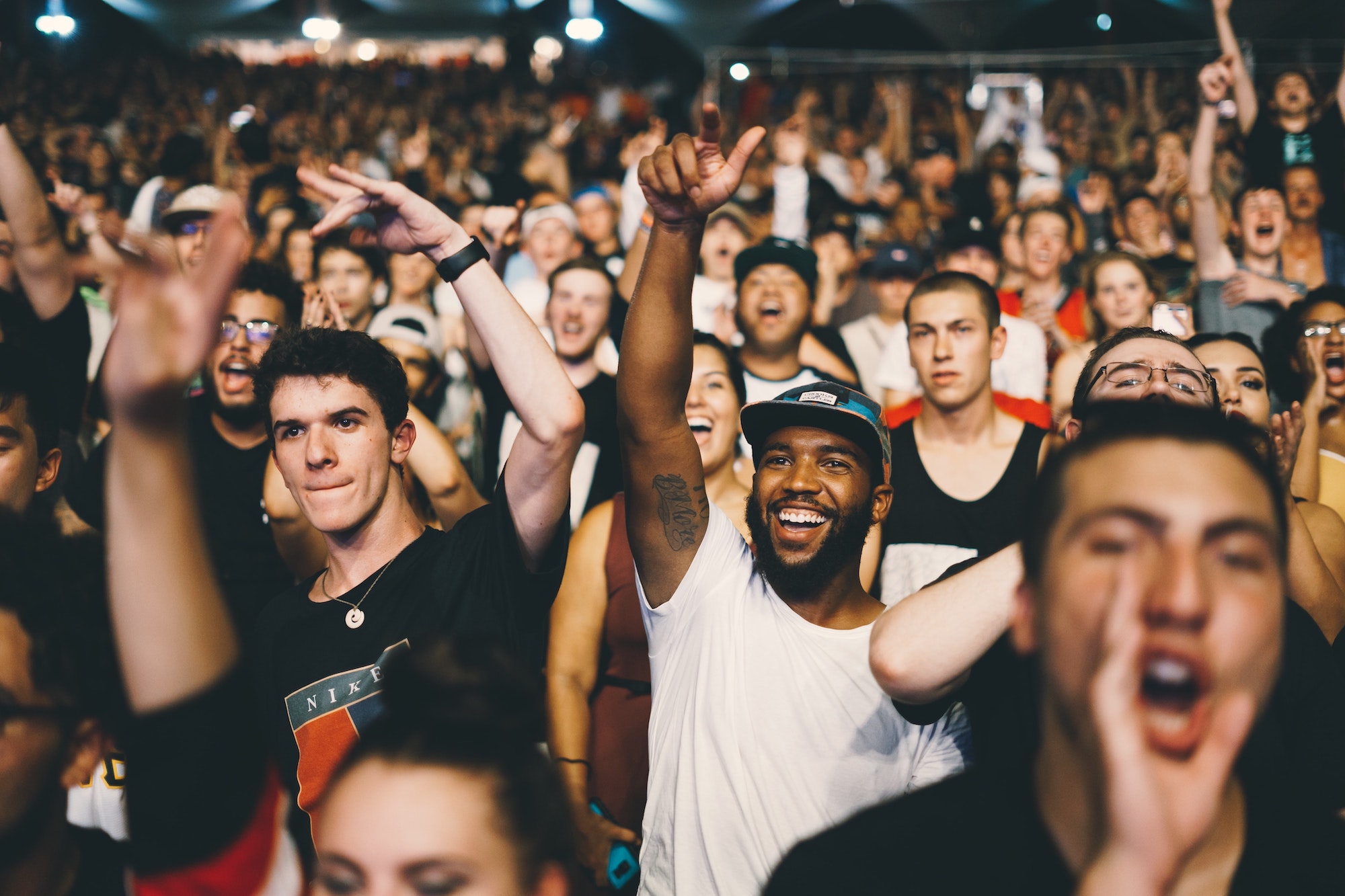
We've all said it:
- I want to track my site subscribers
- I want to try out serverless computing on AWS
- I want to learn about IoC (Infrastructure as Code)
How can I do it all? Here's your chance.
Finally, a tutorial where you learn while actually building something useful.
This tutorial will have you tracking your subscribers in AWS in no time. By the end of this article, you'll have a REST API in AWS that stores subscriber records in a DynamoDB table. You'll also automate the setup of all AWS infrastructure using CloudFormation.
Need proof? My portfolio site uses the APIs shown here to track subscribers.
If you want your own portfolio site, start with my tutorial to setup your portfolio site in under 30 minutes. If you've already setup the portfolio site, I'll show you how to plug in your subcriber API at the end.
So let's get your subscriber API built!
Before you Get Started
You'll need a few things. You may already have some of them:
This tutorial assumes you are comfortable working in the terminal and using Git. Outside of that, the tutorial will walk you through what you need to know.
About the AWS subscribers project
The complete source for the service you'll be setting up can be found on GitHub: aws-subscribers. The project creates a REST API hosted on AWS that will support 2 simple requests:
POST /subscriber
(creates a new subscriber)
{
email: "info@focus.dev"
}
PUT /subscriber/info@focus.dev
(updates subscriber information allowing unsubscribe)
{
email: "info@focus.dev",`
unsubscribeAll: true`
}
The components of the REST API that will be generated by the project can be represented in AWS by the following diagram:
This project serves as a great base for any functionality you may want to add. Here's a quick summary of what the project provides you:
- Automated infrastructure and deployment using AWS SAM and CloudFormation
- Standardized-project structure
- Lambda function coded in TypeScript
- NoSQL database access using DynamoDB
- REST API exposed through API Gateway
Let's get started!
Setting up the Project
Once you have installed the necessary prerequisites you can setup the project with the following terminal commands:
$ mkdir <project-directory>
$ cd <project-directory>
$ sam init --location git@github.com:jorshali/aws-subscribers.git
$ npm install
The starter project will now be available in the project directory you specified.
Create an AWS Profile
This is the most involved part of the tutorial, but it's a one-time process.
The steps below will guide you through the creation of a user with Administrator rights and a local AWS profile for that user. This will allow you to execute the remote commands that fully automate the setup of necessary infrastructure and code deployment.
We'll start by logging into the AWS Console. You will want to search for and select the IAM service. Once in the IAM interface, select Users in the left panel. Here you will want to select Add User.
You will be presented with the Create user steps. Start by providing a valid user name and select Next.
On the next step, select the Add user to group option and click the Create Group button. Search for AdministratorAccess and select the check box to the left. Name the group Administrator. Scroll to the bottom and select Create user group.
You will now be taken back to the user permission options, but you will see your newly created Administrator group. Check the box to the left of your newly created group and select Next.
This will take you to the Review and Create screen. Simply select Create User.
Now that we have our user, we need to generate an access key that will be used locally for running our commands. Back on the Users screen, select the user you just created.
Select the Security Credentials tab and scroll down to the Access Keys section. Here you will want to select the Create access key button.
When presented with Step 1, select the Command Line Interface (CLI) option. Select the "I Understand..." checkbox and select Next.
On Step 2, there's no need to create any description tags so simply select Create Access Key. You will now see the following with your Access Key and Secret Access Key. Leave this page open for the next step.
Now in your terminal, execute the following command:
aws configure --profile aws-subscribers-prod
This will ask you for your AWS Access Key ID and AWS Secret Access Key.
Open back up the page you left open so you can copy and paste those values. Complete the questions as follows:
AWS Access Key ID [None]: <copy and paste the Access Key ID here>
AWS Secret Access Key [None]: <copy and paste the Secret Access Key here>
Default region name [None]: us-west-2
Default output format [None]: json
That's it! The hard part is over. Now let's get this service deployed.
Building the Project
We have to start by telling the AWS CLI that we want to use the profile we just created to deploy the project. For example, in your terminal on a Mac:
$ export AWS_PROFILE=aws-subscribers-prod
Now make sure you are in your project directory in your terminal. Once there, we can build the project with AWS SAM:
$ sam build --beta-features
Note: The beta-features flag is necessary since we are using TypeScript.
Once the build completes, it's time to deploy.
Deploying the Project to AWS
You can now deploy to your account using the profile you selected:
sam deploy --guided
While being guided through the deployment, the defaults are generally recommended. There are only a couple of exceptions. You can customize the Stack Name to something specific to your project. When presented with the following prompt, answer y:
SubscriberFunction may not have authorization defined, Is this okay? [y/N]: y
Once the deployment completes, it will print out 3 results:
Hang onto the WebEndpoint value as this is the URL you will be using to call your Subscriber API.
You can now login and browse your AWS Console to see the various components that were installed. For example, you will find the subscriberFunction
within the Lambda console.
Configuring your personal site (optional)
If you followed my tutorial to setup a portfolio site you can start using your API immediately. In your personal-site-starter
project, open the file src/constants.ts
.
In this file, find the constant SUBSCRIBER_REQUEST_URL
and replace the value with the WebEndpoint value that was output by the SAM deployment.
Save the file and open a terminal in your personal-site-starter
directory. Start your local server by running the following command:
npm run dev
Open your site in your browser at https://localhost:3000. As soon as the landing page opens, put in your email address and click Subscribe Now.
Now open your AWS console and search for DynamoDB. Open it and in the left navigation select Tables > Explore Items. Under Tables select SUBSCRIBER.
Here you will see an entry for your email address!
If everything worked, simply commit these changes and push them to your Git provider. AWS Amplify will automatically redeploy your site with the subscriber API configured.
Running the Service Locally (optional)
You can test your service locally using Docker. You can install Docker here. Once Docker is installed, you can start the project locally with the following commands:
$ sam build
$ sam local start-api --port 8080
Once this is running you can test the API at: http://127.0.0.1:8080/subscriber
If you are testing the personal-site-starter
locally, simply change the value of SUBSCRIBER_REQUEST_URL
in src/constants.ts
to: http://127.0.0.1:8080
Project Organization
This guide is purely focused on getting the Subscriber API up-and-running on AWS quickly. In an upcoming series, I will walk you through more project specifics.
If you want to get started customizing the project right away, here's a quick rundown of the project structure. You can browse this code from the project root in an IDE like VS Code:
template.yml
: the CloudFormation template that defines the service requirements. If you want to customize the REST API URL, DynamoDB table definitions, etc, this is the place to do it.subscriber
: contains the code for thesubscriberFunction
. This is where you will find thepackage.json
dependency definitions, TypeScript files, and general configuration.subscriber/index.ts
: the TypeScript function that handles all/subscriber
requests. You can use this to experiment with your own functionality.
Your service is live on AWS
That's it! You now have an API for capturing subscribers and allowing them to unsubscribe.
If you run into any issues along the way, feel free to ask questions or post issues at: aws-subscribers.
What's next?
In the next post I'll be showing you how to register a domain and connect it through Route 53 in AWS so you can share your site with the world.